How to install Xdebug for XAMPP
A guide focusing on the parts involved, and why they are involved. I’m trying to provide understanding on WHY when you’re trying to get Xdebug working. Sometimes following a guide and just running a few commands on your computer doesn’t teach you much about what’s going on, and if it goes wrong, can be a real pain to recover from, especially if you find yourself needing to uninstall old Xdebug installation attempts. Sometimes the hardest thing is lacking the context for how a program is supposed to work, and where it fits on your computer.
What is Xdebug and why do we want it for our XAMPP setup?
Xdebug is an incredibly useful tool that’s open source and amazing for PHP development, it has a reputation for being difficult to install. This can often mean you’ll have PHP developers and even other types of developers who need to work on a PHP project briefly toughing it out, and not being able to use a debugger, because it’s too much of a headache.
Is it possible to do your PHP development without a debugger? Yes of course. It’s not my favorite way to go. And unless unless you’re an expert already you’ll have to spend a lot of time figuring things out, and printing out variable values.
In my own experience learning PHP and using it to make WordPress Plugins, API endpoints, and simply printing information on the page I found it incredibly helpful to use a debugger and see the variables and to see how the arrays were set up. Seeing if a PHP array has indexes or if it’s using keys is simple in the debug window, but can drive you mad if you’re trying to follow along.
It also helps when it’s not very easy to get to a certain part of your code base. Oftentimes I’ll find myself trying to run var_dump and die() (a trusty combo indeed), or error log to get to the variable I’m trying to read. But in functions that are called very often, or are in a large loop, you’re going to have a hard time getting to that one iteration through the loop, or that one call where the error is showing up. Breaking on errors can save you lot’s of time and mental energy this way.
It’s so much nicer to just push the button press F5 on your code editor and just run the code. For this reason I recommend setting up your debugger on VS Code or on whatever tool you like to use. If you don’t have a proper text editor, or if you’re using an old one, you’re going to have a much harder time, but I imagine VIM or Emacs has a way for you to step through code debugging. But I can’t help you there. I generally debug using Vscode, and I use it just about every day.
So what exactly is required ?
Because you using XAMPP you have Apache installed with the plugins required for it to connect to MYSQL and PHP at least, there are other plugins, but those are the ones you need to run your PHP website and to connect to the database. There are special configuration options you can use that’ll set up Apache to hook into the Zend Xdebug ddl or executible file in order to stop code execution, or provide profiling or whatever you need.
All you need to do to make sure that your text editor debugger is working is to install Xdebug, that is, have the ddl file, or the executible in the right place, with configuration in apache pointing to it. Saying basically “Hey Apache, look at this diretory, that’s where Xdebug’s file is”.
Once that’s ready, Apache will be ready to reach into the zend xdebug module, start up the Xdebug process, and serve it up to your text editor.
As long as you can make sure your text editor’s configured to look at the correct port, your text editor should be able to connect to the Xdebug process, and everything will work, and you’ll be able to breath a sigh of relief mixed with victory.
To get it working in Vscode it’ll look like this.
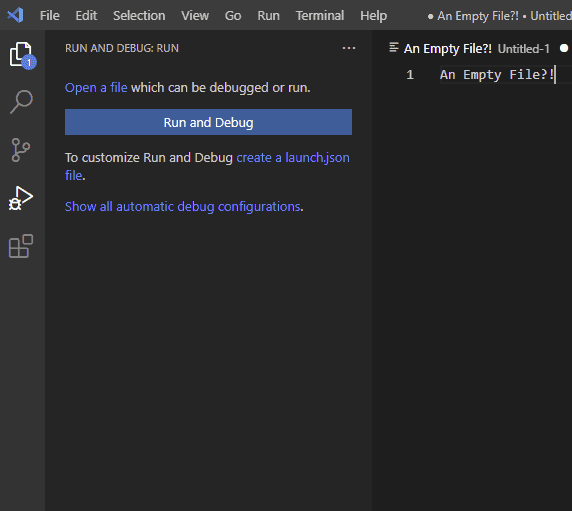
You’ll want to install a PHP debug extension, but once you do you’ll be able to create a default configuration file for your debugger.
Click create a launch.json file, and safe the file it creates for you.
Most likely you will not need to change the default configuration, because it’ll be looking for Port 9000 which is the normal port and probably the easiest one to use. The next thing you’re going to need to do is, you’re going to need to install Xdebug on to your computer. Depending on what kind of computer you’re using the easiest way to do this will vary greatly.
If you’re on Linux and you’ve installed PHP with a package manager like Apt or Yum then there’s probably a command that will automatically install xdebug for you, you’ll need to search for it.
For example:
sudo apt-get install php-xdebug
However you can use an old tool called PECL to install xdebug automatically if you have PHP installed in a different way.
If you’re using Windows there is a Wizard you can use.
And if you’re using anything else, and you’re trying to do it the hard way, you can compile it from it’s source code. It’ll take a long time it’ll require a lot of work it’s just not the way to go if you’re new, and I haven’t had a lot of luck trying it that way.
For Windows:
In order to get the right DDL files, we can follow this link, and download the one we need for our version of PHP installed.
// To get to the php version, you may need to open XAMPP terminal and run
php -v
https://gist.github.com/odan/1abe76d373a9cbb15bed
There is a folder on your computer where XAMPP is installed and an \ext folder inside. That’s where you’re going to put the .dll file then you’ll need to edit the php.ini (configuration) file to activate it. It’ll look something like this.
[XDebug]
zend_extension = C:\xampp\php\ext\php_xdebug-2.5.0-7.1-vc14.dll
xdebug.default_enable=1
xdebug.remote_enable=1
Your PHP ini file will probably have zend_extension configuration commented out, you can uncomment it, or just put in the configuration required.
It’s one more thing you’re going to need you want to set a cookie on your browser using a Chrome extension or an extension for your the browser you’re using or if there’s no extension because you’re using an unusual browser you’ll have to set a cookie yourself it’s a cookie that just says axp bug you’re okay to go and it looks like this
For mac!
For Mac you’re going to need to install it using pickle that’ll probably only work shoot cell thp using Homebrew Ultimately the important thing to know is that you’re trying to install extension to Apache see bug program which is like a bin file for Unix systems or a dll file for Windows and then you’re going to want to open up your code editor and make sure it’s listening on the right Port which is probably 9000
https://xdebug.org/docs/install#pecl
pecl install xdebug
For Apple M1
arch -x86_64 sudo pecl install xdebug
You’ll want to check your php.ini file.
You can find it by looking at your phpinfo() results for a php page and this command.
<?php phpinfo();?>
Or running
php -ini
Once you find your php.ini file, open it up and make sure that these configuration lines are set up. You’ll want to put the path to your own xdebug.so file here.
[XDebug]
zend_extension=/usr/lib/php/20190902/xdebug.so
xdebug.default_enable=1
xdebug.remote_enable=1
Common problems you might run into
If you try to install the wrong version of Xdebug you’re going to get errors and it’s not going to work. You have to make sure that you’re PHP version that you’re using an Apache matches the PHP version you’re using in XAMPP.
Port 9000 might be in use! If it’s in use you’re going to have to change the configuration file to use a different port. Or close down whatever program is using up Port 9000.
Multiple PHP insistence on your computer. This can make it confusing. You might install Xdebug on one, but if it’s not the right one there’s nothing for your Code editor to latch on to. This can happen if you need a few versions of PHP installed, and you use a script to switch between them. Or you have PHP installed in a few ways. Where the terminal has one version available, and XAMPP has it’s own version that’s seperate. Installing Xdebug on one will not help you with the other, especially if they are separate versions.
I’m hoping that this guide clears things up for you. I hope it helps you get your debugging set up working.